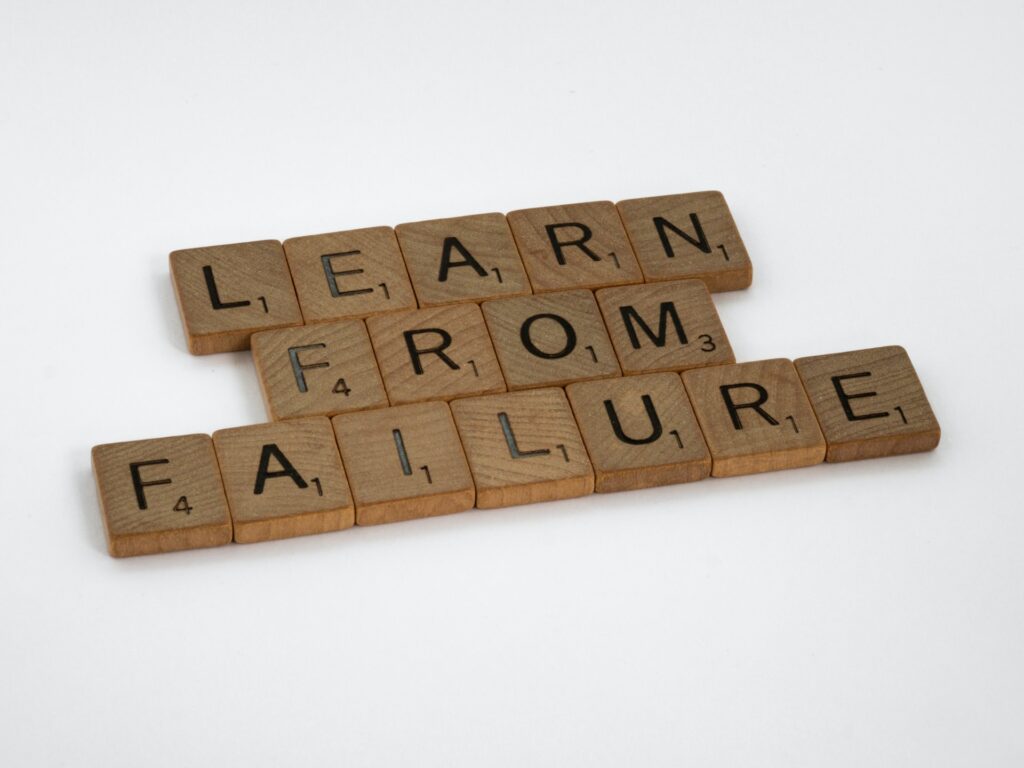
If you’re a junior React Native developer, chances are you’re already familiar with the basics of the library and have started to explore more complex features, such as the useEffect
hook. However, as with any powerful API, it’s not without its pitfalls.
This article will guide you through the common mistakes React beginners make when using useEffect
, and show you how to avoid them.
What is useEffect?
React’s useEffect
hook allows you to perform side effects in function components.
A “side effect” in programming can be defined as an operation that modifies some state outside its local environment. In the context of React, side effects could include fetching data, manually changing the DOM, setting up a subscription, or even logging to the console. These operations interact with the world outside the function/component they are used in.
useEffect
is a replacement for several lifecycle methods in class components, including componentDidMount
, componentDidUpdate
, and componentWillUnmount
.
useEffect
tells React that your component needs to do something after render. After learning how to use this hook effectively, you avoid all the performance pitfalls that can degrade the experience for your users.
In this article, we’ll cover the most common mistakes made with useEffect
and how to avoid them.
Common useEffect Mistakes
The useEffect
hook can be a bit tricky to get the hang of, especially for developers who are new to React.
Here are some common pitfalls to be aware of:
Mistake 1: Not Using the Dependency Array
useEffect(() => {
console.log('This runs after every render');
});
The dependency array is a crucial aspect of useEffect
that is often overlooked. If you leave it out, your effect will run after every render, which can lead to performance issues.
Instead, you should pass an array of dependencies to useEffect
which determines when the effect should run. If the values in this array change between renders, the effect will run again.
// This runs once when the component mounts, and again if `dependency` changes
useEffect(() => {
console.log('Hello');
}, [dependency]);
Mistake 2: Putting Functions Inside useEffect
useEffect(() => {
function fetchData() {
// Fetch data here
}
fetchData();
}, []);
Defining functions inside useEffect
that aren’t part of the effect can lead to unexpected behavior. Instead, define your functions outside of useEffect
and call them inside your effect.
function fetchData() {
// Fetch data here
}
useEffect(() => {
fetchData();
}, []);
Mistake 3: Forgetting to Handle Component Unmounting
useEffect(() => {
const subscription = someAPI.subscribe();
// Forgot to unsubscribe! Memory leak!
}, []);
When you set up a subscription or event listener in useEffect
, you should always clean it up when the component unmounts to prevent memory leaks. This can be done by returning a function from useEffect
.
useEffect(() => {
const subscription = someAPI.subscribe();
// Unsubscribe when the component unmounts
return () => {
subscription.unsubscribe();
};
}, []);
Mistake 4: Overusing useEffect
useEffect(() => {
setFormErrors(validateForm(formValues));
}, [formValues]);
In this example, the developer is using useEffect
to validate form values each time they change and set the form errors accordingly. While this might seem like a good idea, it can lead to unnecessary renders and can make the form feel less responsive to the user.
useEffect
is a powerful tool for handling side effects in your components, but it should not be used for all state changes. In fact, overusing useEffect
can lead to performance issues and unexpected behavior in your app.
Instead, a better approach could be to validate the form values when the form is submitted or when a user stops typing, rather than on every change. This can be done using the useState
and useCallback
hooks :
const [formErrors, setFormErrors] = useState({});
const validateAndSetErrors = useCallback(() => {
setFormErrors(validateForm(formValues));
}, [formValues]);
// Then, call validateAndSetErrors when the form is submitted or when user stops typing
In this revised example, form validation happens when the form is submitted or when a user stops typing, rather than on every form value change.
useEffect
is best used for handling side effects that need to occur after render, such as fetching data, setting up subscriptions, or interacting with browser APIs. Not every state change needs to trigger an effect. Sometimes, other hooks like useState
and useCallback
are more appropriate. Choose wisely!
Mistake 5: Deriving Data using useEffect
const [sum, setSum] = useState(0)
useEffect(() => {
let sum = 0;
for (let i = 0; i < array.length; i++) {
sum += array[i];
}
setSum(sum);
}, [array]);
useEffect
is designed for side effects – operations that need to occur after render and that don’t directly influence the component’s render output. A common mistake is using useEffect
for calculations or logic that could be done directly in the render method or elsewhere in your component.
In the example above, a sum is being calculated within useEffect
when the array prop changes, and then set to state, triggering a re-render. This is unnecessary and could be done more efficiently without useEffect
.
Instead, this sum could be calculated directly in the render method, or better yet, memoized with the useMemo
hook to avoid unnecessary calculations on each render:
const sum = useMemo(() => {
return array.reduce((acc, value) => acc + value, 0);
}, [array]);
In this revised example, useMemo
calculates the sum only when the array
changes, avoiding unnecessary calculations and re-renders, and keeping your component performance optimized.
useEffect
is primarily for dealing with side effects. It’s not a catch-all place to put your logic. If you find yourself putting a lot of unrelated logic in your effects, it might be time to refactor your component or consider other hooks.
Frequently Asked Questions
In this section, we’ll answer some of the most common questions about useEffect
and the common mistakes discussed in this article.
What is a side effect in React?
A side effect in React is an operation that interacts with something outside of the component’s local environment. This could be anything from updating the DOM, fetching data from an API, setting up subscriptions, or manually changing the document title. Side effects usually occur after a component’s render has finished.
When should I use useEffect
?
useEffect
should be used for side effects that need to occur after a component has rendered or when certain values have changed. This could include operations like fetching data, interacting with browser APIs, setting up subscriptions, or any operation that doesn’t directly influence the component’s render output.
What is the dependency array in useEffect
?
The dependency array in useEffect
is a list of values that the effect depends on. If any of these values change between renders, the effect will run again. If the array is empty ([]
), the effect will only run once after the initial render (similar to componentDidMount
in class components).
Why does useEffect
cause an infinite loop in my component?
useEffect
can cause an infinite loop if it’s updating a state that’s included in its dependency array. Each state update triggers a re-render, which in turn triggers the effect again, leading to an infinite loop. To avoid this, ensure that you’re not causing unnecessary state updates within your effects.
How can I avoid common mistakes when using useEffect
?
Understanding the purpose of useEffect
is key to avoiding common mistakes. Remember that useEffect
is for side effects that need to occur after render. Avoid overusing useEffect
for tasks that could be done elsewhere in your component or with other hooks. Always handle component unmounting to avoid memory leaks and unexpected behavior.
What are some alternatives to useEffect
for managing state in React?
React provides several other hooks for managing state, including useState
for local state, useContext
for shared state, useReducer
for complex state logic, and useMemo
and useCallback
for performance optimization.
Why is it important to handle component unmounting in useEffect
?
Handling component unmounting in useEffect
is important to clean up any subscriptions, timers, or other side effects that were set up in the effect. Failing to do so can lead to memory leaks, unexpected behavior, or errors.
Can I use useEffect
in functional components only?
Yes, useEffect
is a hook, and hooks can only be used in functional components. Class components have similar lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
for handling side effects.
How does useEffect
differ from other lifecycle methods in class components?
useEffect
unifies several lifecycle methods from class components. It runs after every render, similar to componentDidUpdate
, but if you pass an empty array ([]
) as the second argument, it runs only once after the initial render, similar to componentDidMount
. You can also return a cleanup function from useEffect
to handle component unmounting, similar to componentWillUnmount
.
Can I use multiple useEffect
hooks in a single component?
Yes, you can use multiple useEffect
hooks in a single component. This is often done to separate unrelated logic into different effects. Each useEffect
hook can have its own set of dependencies, which allows you to control how often different effects run. Using multiple useEffect
hooks can make your code more modular, easier to test, and easier to understand.
Wrapping up
Working with React’s useEffect
hook can be a bit daunting, especially if you’re just starting out with React.
It’s a powerful tool, but like any tool, it requires a good understanding of React lifecycle and rendering concepts, and careful handling to be used effectively.
In this article, we’ve explored five common mistakes that React beginners often make when using useEffect
:
- They do not use the dependency array
- They declare functions inside
useEffect
- They forget to handle component unmounting
- They overuse
useEffect
- They compute data derived from state using
useEffect
So now you know these pitfalls and you understand why they can be problematic.
But remember, the journey of learning never ends. As you gain more experience and encounter more complex scenarios, you’ll undoubtedly discover new challenges and solutions in your work with React and useEffect
.
Thank you for reading, I hope you learned something and looking forward to seeing you in the next post!
To further your understanding of React Native, have a look at some of our other articles:
- 7 Essential JavaScript Concepts for React Native Beginners
- Debugging React Native apps like a Pro in 2023
- Getting Started With React : Your First Step Towards Mastering React Native
- How Long Does it Take to Learn React Native?
- React Native Best Practices in 2023
Further Resources
If you want to dive deeper into useEffect
and React in general, here are some additional resources I can recommand :
- React Hooks API Reference: This is the official React documentation for Hooks, including
useEffect
. It provides detailed explanations and examples. - A Complete Guide to useEffect: This comprehensive guide by Dan Abramov, one of the creators of React, provides a deep dive into
useEffect
. - React Native Documentation: The official React Native documentation is a great resource for learning more about React Native development.
- React Native Blog: This blog features articles on a variety of topics related to React Native, including best practices, updates, and tutorials.