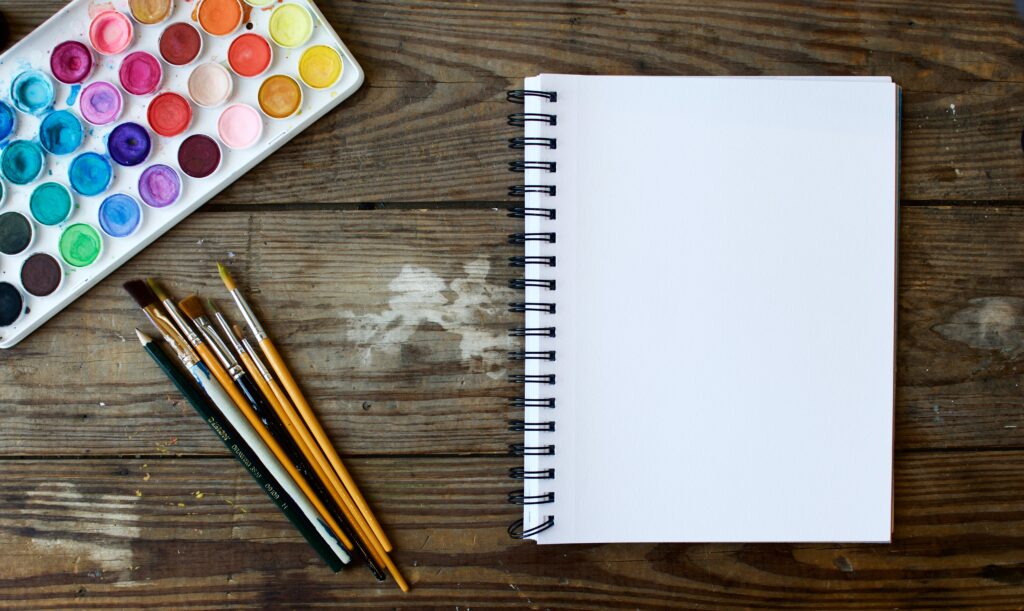
Welcome to React Native Central – your go-to resource for everything related to React Native! Whether you’re a seasoned developer or just starting your React Native journey, understanding how to style your apps is paramount for creating intuitive, visually appealing apps that users love.
In this article, titled “The Beginner’s Guide to Styling in React Native”, we’ll guide you through the basics of styling in React Native. We’ll uncover why styling is important and how it impacts the user experience. We’ll also go through into the differences between inline styles and stylesheets, helping you discern when to use each approach.
What is Styling in React Native?
Styling in React Native is not just about making your app look good—it’s about defining the visual identity of your application and improving user experience. Similar to styling in web-based CSS, styling in React Native controls the layout and appearance of your app’s user interface.
In React Native, we use JavaScript to write our stylesheets. This may seem unusual if you’re coming from a CSS background, but the advantage is that everything in your app, including styles, is unified under one language. The core concept involves defining styles as JavaScript objects.
There are two primary ways to handle styles in a React Native app:
- Inline Styles: Inline styles are defined directly in the render method as a JavaScript object within the style prop. They are quick to write and provide immediate visual feedback.
- Stylesheet: A StyleSheet is a JavaScript abstraction similar to CSS StyleSheets. Styles in StyleSheet live separately from the component, which can improve readability and reusability of styles across components.
Why is Styling Important in React Native?
Styling in React Native plays a pivotal role in the overall user experience of your mobile app. But why is it so essential? Here are a few reasons:
- Aesthetics: First and foremost, styling is what makes your app aesthetically pleasing. A well-styled app appeals to users, encouraging them to interact with the app more frequently and spend more time in it.
- Branding: Styling helps you incorporate brand elements like colors, typography, and logos consistently throughout your app. This not only enhances brand recognition but also contributes to a cohesive look and feel.
- Usability: Good styling goes beyond aesthetics. It contributes to the usability and accessibility of an app. Considerations like text size and color contrast make your app more accessible to a wide range of users, including those with visual impairments.
- Consistency: With React Native’s styling, you can ensure a consistent appearance across different devices and platforms (iOS and Android).
- Feedback and Interaction: Styling aids in providing feedback to user actions. Visual cues like button color change on press help users understand the result of their actions, creating a more interactive experience.
- Layout and Spacing: Styling is also responsible for the layout of elements on the screen, which affects how users navigate your app. Good use of space, alignment, and positioning can make your app easier to use.
In conclusion, effective styling is a powerful tool that can greatly enhance the functionality and user experience of your React Native applications. By investing time in learning about styling in React Native, you are investing in the success of your apps.
For the sake of this guide, we will cover the two main approaches to Styling that comes out-of-box when creating a new React Native project: Inline Styles and Stylesheet.
While both methods have their pros and cons, it’s important to choose the one that best suits your specific use case. In the next sections, we will discuss how to work with both inline styles and stylesheets.
Working with Inline Styles
Inline styles are a quick way to apply styles directly to a component. They’re written as a JavaScript object within the style prop of the component you’re styling.
Here’s a simple example:
<View style={{ backgroundColor: 'blue', padding: 10 }}>
<Text style={{ color: 'white', fontSize: 20 }}>
Hello, React Native!
</Text>
</View>
In this example, the View
component has a blue background and padding of 10 units. The Text
component within the view has white text and a font size of 20 units.
Benefits of Inline Styles
- Rapid prototyping: Inline styles are a fast and easy way to experiment with styles on the fly.
- Small components: For components with minimal styling needs, inline styles can be a quick and straightforward solution.
- Dynamic styles: If a style depends on a condition or changes frequently, inline styles are a good option as they can be dynamically calculated.
Drawbacks of Inline Styles
- Lack of reusability: If the same styles are used across multiple components, you’ll end up rewriting the same styles.
- Harder to maintain: For complex components or applications, inline styles can get messy, making them harder to read, manage, and debug.
- Performance: Although minimal, inline styles can lead to slower performance compared to stylesheets as a new style object is created each render.
Overall, while inline styles can be quick and easy, they’re best suited for smaller, less complex styling tasks. For more extensive styling needs, it might be more beneficial to use stylesheets, which we’ll explore in the next section.
Working with Stylesheets
Stylesheets in React Native allow you to handle your app’s styling in a more organized and maintainable manner. A Stylesheet is a JavaScript abstraction similar to CSS stylesheets, where you define all your styles in one place and reference them in your components.
Here’s an example of how to use a Stylesheet in React Native :
import { StyleSheet, Text, View } from 'react-native';
export default function App() {
return (
<View style={styles.container}>
<Text style={styles.text}>
Hello, React Native!
</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
backgroundColor: 'blue',
padding: 10,
},
text: {
color: 'white',
fontSize: 20,
},
});
In this example, we define a styles
object using StyleSheet.create()
, where we define our styles. We then apply these styles to our components using style={styles.styleName}
.
Benefits of Stylesheets
- Organization: Stylesheets help keep your styles separate from your component logic. This way the code is cleaner and easier to read.
- Reusability: With Stylesheets, you can define a style once and use it across multiple components, promoting DRY (Don’t Repeat Yourself) principles.
- Performance: Stylesheets can provide a slight performance benefit over inline styles because the style object is created once and reused across renders.
Drawbacks of Stylesheets
- Increased Complexity: For very simple components or prototypes, creating a stylesheet may add unnecessary complexity.
- Less Flexibility: Stylesheets are less flexible for dynamic styles that depend on state or props.
- The overhead of switching between the style object and the component can slow down development in some cases.
Understanding when to use inline styles versus stylesheets is an essential part of mastering React Native styling. In the next section, we’ll go through some best practices to further improve your React Native styling skills.
Best Practices for Styling in React Native
Implementing best practices can significantly enhance the performance and maintainability of your app. Here are some best practices to consider, especially when working in a team:
- Reuse Styles: Make use of stylesheets to define common styles and reuse them across components. This leads to cleaner code and easier maintenance.
- Organize Stylesheets: Group related styles together in your stylesheet. This could mean grouping styles by component or by purpose (e.g., typography, colors, layout).
- Use Descriptive Names: Style names should be self-descriptive. Someone reading your code should get a sense of what a style does just by reading its name.
- Avoid Magic Numbers: Avoid using arbitrary numbers in your styles. Instead, define a set of standard spacing and sizing values and use them consistently across your app.
- Use Responsive Units: Consider different screen sizes when styling your app. Make use of percentages and responsive units to ensure your app looks good across a range of devices.
- Leverage Platform-Specific Styles: React Native allows you to define platform-specific styles. Use this feature to fine-tune your app’s appearance on different platforms (iOS and Android).
- Take Advantage of External Libraries: Don’t hesitate to use external styling or theme libraries, such as styled-components or react-native-paper, to speed up development and improve code quality.
- Accessibility: Consider accessibility in your styles. Use sufficient color contrast, readable font sizes, and clear touch targets to ensure your app is accessible to all users.
Conclusion
In this guide, we’ve explored the fundamentals of styling in React Native, including inline styles and stylesheets. We’ve also covered some best practices that can elevate your styling game and make your apps more efficient, maintainable, and user-friendly.
Remember, like any skill, mastering styling in React Native takes time and practice. Don’t hesitate to experiment, explore different approaches, and learn from your experiences.
I hope you found this guide helpful! For more React Native resources, tips, and tutorials, be sure to stay tuned to React Native Central.
Looking forward to seeing you in the next post!
To further your understanding of React Native, have a look at some of our other articles: