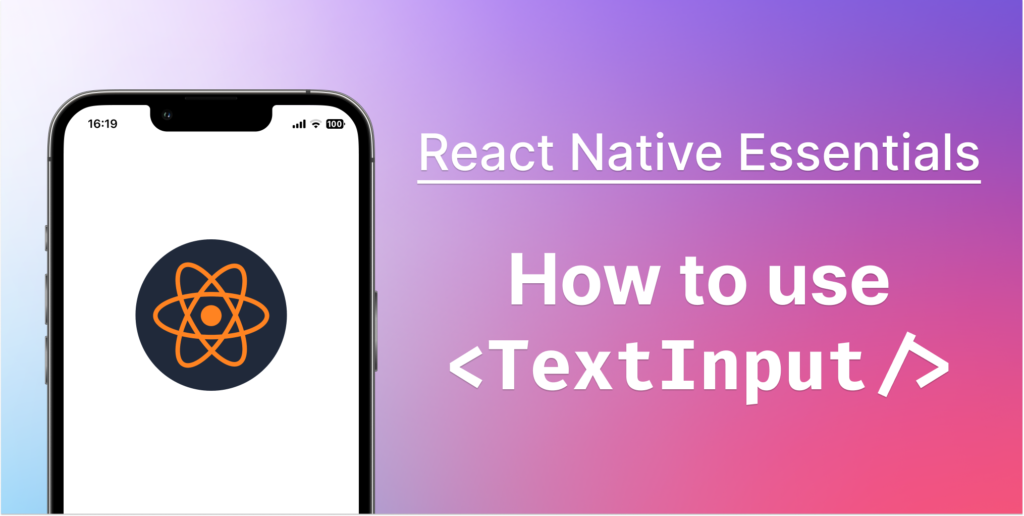
In this article of our series “React Native Essentials”, today we’ll have a look TextInput
component from React Native.
Whether you’re a beginner trying to grasp the basics or a seasoned developer looking to brush up your knowledge, this guide offers a broad perspective on TextInput usage. By the end of it, you’ll not only understand how to implement and customize TextInput but also handle the data obtained from it effectively.
TextInput: What Is It?
TextInput is a component from the React Native library, that let users type text into your app. It’s essentially the React Native equivalent of the HTML ‘input’ element.
This component forms the cornerstone of any application that requires user interaction, such as entering data, filling forms, or typing messages.
Why Use TextInput in React Native?
- User Input: The most fundamental use of TextInput is to gather user input. Whether it’s a login form, a search bar, or a chatbox, TextInput fields facilitate the flow of information from the user to the application.
- Flexibility: TextInput in React Native is highly customizable. You can adjust aspects such as the keyboard type, placeholder text, text color, padding, borders, and much more. This flexibility allows you to design a TextInput field that seamlessly integrates with your app’s overall design and functionality.
- Control: Beyond its customization capabilities, TextInput also provides control over the user input. You can restrict the type and length of input, and even implement real-time validation as the user types.
- Event Handling: TextInput fields in React Native are not just passive elements waiting for user input. They are interactive fields that can trigger events based on user actions. For example, you can set an action to occur when a user starts or stops typing, or when they submit a form.
- Enhanced User Experience: By using TextInput effectively, you can greatly enhance the user experience. Autocorrection, multiline input, and secure text entry for sensitive data are among the many features that make TextInput a crucial component in creating user-friendly mobile apps.
In the next section, we’ll take a step-by-step approach to implementing TextInput in a React Native codebase.
Setting Up TextInput
Here is a step-by-step guide to implementing a basic TextInput field:
import React, { useState } from 'react';
import { TextInput, View } from 'react-native';
const MyTextInput = () => {
const [value, setValue] = useState('');
return (
<View>
<TextInput
style={{ height: 40, borderColor: 'gray', borderWidth: 1 }}
onChangeText={text => setValue(text)}
value={value}
/>
</View>
);
};
export default MyTextInput;
- Import Necessary Components: The first step is to import the necessary components from the ‘react’ and ‘react-native’ libraries. We import
React
anduseState
from ‘react’, andTextInput
andView
from ‘react-native’. - Initialize State: We use the
useState
hook to initialize the state for our TextInput field. In this example, we’re creating a state called ‘value’ that will store the user’s input. - Return TextInput Component: Inside the component’s return statement, we nest the TextInput field inside a View component.
- Styling TextInput: Using the
style
prop, we set the height of our TextInput field to 40 and the border color to gray with a border width of 1. - Handle Text Change: With the
onChangeText
prop, we handle the change in text input. The function insideonChangeText
updates the ‘value’ state each time the user types or deletes something. - Value Prop: The
value
prop sets the current value of the TextInput field to our ‘value’ state. This establishes a single source of truth for our TextInput value and allows React to control the rendered output.
Customizing TextInput
Let’s go through a few TextInput props that you can use to make your TextInput fields more engaging :
import React, { useState } from 'react';
import { TextInput, View } from 'react-native';
const MyTextInput = () => {
const [value, setValue] = useState('');
return (
<View>
<TextInput
style={{ height: 40, borderColor: 'gray', borderWidth: 1 }}
onChangeText={text => setValue(text)}
value={value}
placeholder="Enter your text here"
keyboardType="email-address"
secureTextEntry
maxLength={40}
/>
</View>
);
};
export default MyTextInput;
- Placeholder: This property allows you to set placeholder text in your TextInput field, providing a hint to users about what they should enter. In the above example, we’ve set the placeholder text as “Enter your text here”.
- KeyboardType: This property adjusts the keyboard type that appears when a user taps on the TextInput. Setting
keyboardType="email-address"
will display a keyboard optimized for email input. - SecureTextEntry: This property hides the input text, which is particularly useful for password fields. When
secureTextEntry
is enabled, any text inputted by the user will be masked. - MaxLength: This property sets a limit on the number of characters that can be entered in a TextInput field. In the above example, the user can input a maximum of 40 characters.
- Multiline: This property allows the TextInput field to expand and accommodate multiple lines of input. Setting
multiline
totrue
is especially useful when users are expected to enter more than one line of text.
These are just a few of the properties that TextInput offers. Make sure to read the official docs of TextInput to learn about all the possibilities of this component.
In the next section, let’s have a look into how to handle the data entered into TextInput fields effectively.
Handling TextInput Data
React Native provides several techniques to effectively handle TextInput data, including validation, state management, and controlled components. Let’s look at an example where we handle TextInput data effectively.
import React, { useState } from 'react';
import { TextInput, View, Button, Alert } from 'react-native';
const MyTextInput = () => {
const [value, setValue] = useState('');
const handleSubmit = () => {
if(value.trim() === '') {
Alert.alert('Error', 'Input field cannot be empty');
} else {
Alert.alert('Success', `You entered: ${value}`);
setValue('');
}
};
return (
<View>
<TextInput
style={{ height: 40, borderColor: 'gray', borderWidth: 1, marginBottom: 20 }}
onChangeText={text => setValue(text)}
value={value}
placeholder="Enter your text here"
/>
<Button title="Submit" onPress={handleSubmit} />
</View>
);
};
export default MyTextInput;
In this example, we have added a button that handles the submission of the TextInput data:
- Create a Submit Handler: We define a function called
handleSubmit
. This function checks if the input field is empty when the submit button is pressed. If the field is empty, an error message is displayed; if not, a success message is displayed with the entered text. - Resetting the TextInput Value: After a successful submission, we reset the ‘value’ state to an empty string. This clears the TextInput field, preparing it for a new input.
Remember that validation is not limited to checking if the field is empty. You can use regex patterns to check the format of the entered text, such as email formats, phone number formats, etc.
Best Practices when Using TextInput
To ensure optimal usage of TextInput in React Native, it’s crucial to follow some best practices:
- Controlled vs Uncontrolled TextInput: Developers often struggle to decide whether to use controlled or uncontrolled components. In controlled components, the state of the component is controlled by React, while in uncontrolled components, they maintain their own state. As a standard practice, prefer controlled components. This approach allows React to control the form’s state, providing a more predictable behavior and making state management easier to orchestrate.
- Provide User Feedback: Always aim to provide users with visual feedback when interacting with TextInput. Highlighting the active field, showing validation error messages instantly, or indicating successful input can greatly enhance the user experience.
- Handle Keyboard Interactions Properly: Ensuring the keyboard does not block input fields or dismisses when it should is an integral part of user interaction. A simple solution is to use React Native’s
KeyboardAvoidingView
or third-party libraries such asreact-native-keyboard-aware-scroll-view
. These tools adjust the view when the keyboard is active to ensure the TextInput field remains visible in the shrunk viewport. - Optimize for Performance: Prevent unnecessary re-renders when handling text inputs to keep your app running smoothly. Consider using debouncing techniques (limiting the rate at which a function can fire) to prevent unnecessary re-renders.
- Ensure Accessibility: Your TextInput fields should be accessible to all users. This involves using a sufficient contrast for text and borders, providing clear and understandable error messages, and ensuring compatibility with screen readers.
- Managing multiple TextInput fields: If you have multiple TextInput fields, managing their states can become complex. One way to handle this is by using a single state object to store all TextInput values.
These best practices can significantly enhance your proficiency with TextInput in React Native, leading to more interactive and user-friendly mobile applications.
Frequently Asked Questions
In this section, we’ll address some of the common questions that developers often have about using TextInput in React Native.
Can I use TextInput for password fields?
Yes, React Native’s TextInput has a prop called secureTextEntry
that can be used for password fields. When set to true
, this prop obscures the text entered into the TextInput, similar to an HTML password field.
How can I change the keyboard type for TextInput?
You can change the keyboard type for TextInput using the keyboardType
prop. React Native supports several keyboard types like default
, numeric
, email-address
, and more.
Can I limit the number of characters in TextInput?
Yes, you can limit the number of characters using the maxLength
prop. This prop takes a number that specifies the maximum number of characters the TextInput can accept.
How do I autofocus on a TextInput field when a screen loads?
You can use the autoFocus
prop to automatically focus the TextInput when a screen component is mounted. Set autoFocus={true}
to enable this behavior.
Can I style TextInput in React Native?
Yes, you can customize the style of TextInput using the style
prop. This allows you to control properties such as color
, fontSize
, height
, width
, and more.
How can I clear the value of TextInput?
You can clear the value of TextInput by changing the state variable that’s controlling it. You can either use a button that clears the state or implement it in any other function as per your app’s needs.
Wrapping up
In this article, we’ve taken a deep dive into what TextInput is, why it’s important, how to set it up, customize it, and handle data entered into it. We’ve also looked at common issues faced while working with TextInput and their solutions, and laid out some best practices for using TextInput.
If you found this guide helpful and want to learn more about other React Native components, stay tuned to React Native Central, your hub for everything related to React Native.
Looking forward to seeing you in the next post!
To further your understanding of React Native, have a look at some of our other articles: