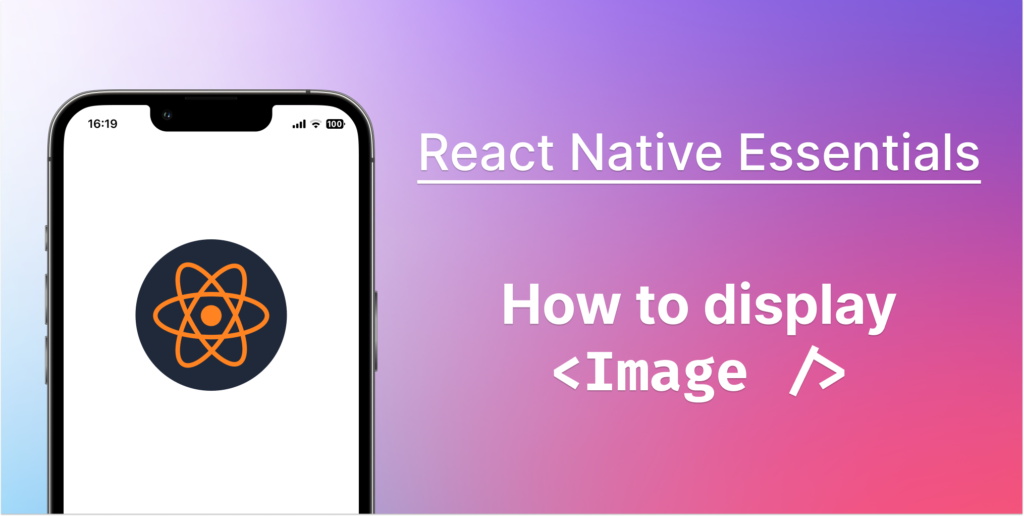
Introduction
Let me share a personal anecdote. A few years back, I was working on a React Native application for a retail client. The application was functional and served its purpose well, but the client reported that their users found it dull and unattractive. The culprit? We had underutilized images in the app, leading to a visual experience that really wasn’t enticing. We decided to step up our game and after consulting with a Product Designer, he gave us a lot more illustrations that we used make the overall user interface more engaging and intuitive. The result? User engagement skyrocketed, and the app received great reviews for its improved aesthetics!
Through this article, I aim to guide you on a journey of mastering one of the key aspects of app development in React Native – displaying images like a Pro. Whether you’re a seasoned developer or a beginner just dipping your toes into the world of React Native, I am sure that you’ll find this guide valuable.
Importance of Images in Apps
Imagine using an app without any images – sounds pretty dull, right? Indeed, it is. Images are the lifeblood of any application. They enhance the visual appeal, aid in communication, and enrich user experience.
I recall one of my projects – an e-commerce app. It was technically sound and functionally complete. However, it lacked the visual appeal due to a minimal use of images. We decided to revamp the design, and started by adding high-quality images for every product. The transformation was incredible! Not only did the app look more lively and inviting, but it also saw a considerable increase in user engagement and retention.
Displaying Images in React Native: A Step-By-Step Guide
Now that we’ve laid the groundwork with the essential concepts, let’s get our hands dirty and start displaying some images in our React Native application. We will follow this process step-by-step to ensure a smooth and comprehensive learning experience. Let’s get started!
Installing Required Packages
Thankfully, React Native comes with the Image
component out of the box, so we don’t need any extra installations for basic image rendering! However, to ensure you have the latest version of React Native, use the following command in your terminal:
npm install react-native@latest
Image Component: A Deep Dive
React Native’s Image
component is the key component of the React Native Trifecta of base elements <View /> <Text /> <Image />
. It’s a versatile component that supports both network images (those that are hosted on a remote server) and local images (those that are bundled with the application).
Here’s a simple example of how you can use the Image
component:
import { Image } from 'react-native';
<Image source={{ uri: 'https://your-image-url' }} style={{ width: 100, height: 100 }} />
This code block pulls an image from a remote URL and displays it with a width and height of 100 pixels. Simple, right? But there’s so much more you can do!
In the next section, we’ll explore how to deal with different image types and sources.
Dealing with Different Image Types and Sources
Now that you’ve got a grasp on loading and displaying images in React Native, let’s dig a little deeper. Just like in web development, we encounter different types of images while building a mobile app, like JPEG, PNG, GIF, and more. Furthermore, these images can be stored either locally in our app or hosted on a remote server. Let’s explore how we can deal with these different image types and sources.
Handling Different Image Types
React Native’s Image
component supports a range of popular image types out of the box. These include JPEG, PNG, GIF, BMP, WebP on Android, and more. Here’s an interesting thing: GIF and WebP images can also be animated!
To display different types of images, you just need to ensure that the source prop points to the correct file, be it local or network. React Native will handle the rest.
Different Image Sources
Images can either be stored locally in your project or hosted on a remote server.
Local Images
Local images are the ones that are bundled with your app. When displaying these, React Native takes care of loading them in the most performant way. To display a local image, you can simply require it directly into the source prop as follows:
<Image source={require('./path-to-your-image.png')} />
Remember, for local images, always use the require
statement.
In one of my projects, we had a lot of static content that needed to be displayed. To optimize the performance, we decided to bundle these images with the app. This decision greatly improved the app’s load times and overall performance.
Network Images
Network images, on the other hand, are fetched from a remote server. This is common when you’re fetching data from an API that includes image URLs. We need to fetch them using their URL. Here’s how you can display a network image:
<Image source={{ uri: 'https://your-image-url.png' }} />
The key difference here is that for network images, you need to use the uri
tag in the source prop.
Remember to handle errors and loading states when dealing with network images as network requests can fail or take time.
My first encounter with network images was quite a learning curve. I was working on an app that fetched a lot of data from a server, including images. The first versions of the app were slow and often crashed. After learning about error handling and loading states, the app became much more stable and responsive.
Advanced Topics
We have covered the basics and you’re now able to display various types of images in React Native. However, there are additional advanced topics that can elevate the performance and user experience of your application. In this section, we’ll discuss performance and optimization techniques, and how to handle network images.
Performance and Optimization Techniques
One of the main challenges when displaying images in an application is ensuring that the process is efficient and doesn’t degrade the app’s performance. This is where optimization techniques come into play.
Resizing Images
You can use the resizeMode
prop of the Image
component to determine how your image should be resized when the frame doesn’t match the raw image dimensions. It accepts cover
, contain
, stretch
, repeat
, center
as values. Each mode handles the image resizing differently, so select the one that best suits your needs.
<Image source={{ uri: 'https://your-image-url.png' }} resizeMode="cover" />
Caching Images
Caching is another important technique to optimize image loading times. It stores images locally after they’re loaded for the first time, which significantly improves loading times in subsequent renders. Luckily, React Native handles caching for us automatically in most cases. However, for network images, it can be useful to use a library like react-native-fast-image
, which provides more control over image caching.
import FastImage from 'react-native-fast-image'
<FastImage
source={{ uri: 'https://your-image-url.png', priority: FastImage.priority.high }}
resizeMode={FastImage.resizeMode.contain}
/>
Handling Network Images
As we know, network images are fetched from a remote server. While this allows us to display dynamic content, it also poses challenges like loading times and handling failures.
Loading Indicators
When fetching an image from a network, it’s good practice to show a loading indicator. This can be easily done by using React Native’s ActivityIndicator
component in conjunction with the Image
component’s onLoadStart
and onLoadEnd
event handlers.
import React, { useState } from 'react';
import { ActivityIndicator, View, Image } from 'react-native';
const ImageView = () => {
const [isLoading, setIsLoading] = useState(false);
return (
<View>
{isLoading && <ActivityIndicator size="large" color="#0000ff" />}
<Image
source={{ uri: 'https://your-image-url.jpg' }}
onLoadStart={() => setIsLoading(true)}
onLoadEnd={() => setIsLoading(false)}
/>
</View>
);
};
This ImageView component shows an ActivityIndicator
when the image is loading.
onLoadStart
is triggered when the image starts loading, which sets isLoading
to true
and shows the ActivityIndicator
. onLoadEnd
is triggered when the image finishes loading, which sets isLoading
to false
and hides the ActivityIndicator
.
Error Handling
The Image
component also provides the onError
event handler, which is called when there’s an error loading the image. You can use this to display an error message or a fallback image.
import React, { useState } from 'react';
import { View, Image, Text } from 'react-native';
const ImageView = () => {
const [isError, setIsError] = useState(false);
return (
<View>
{isError ? (
<Text>Failed to load the image</Text>
) : (
<Image
source={{ uri: 'https://your-image-url.jpg' }}
onError={() => setIsError(true)}
/>
)}
</View>
);
};
In this code, onError
is triggered when there’s an error loading the image, which sets isError
to true
and displays the error message.
I remember working on a news app that heavily relied on network images. Initially, we didn’t include error handling. However, we quickly realized its importance when some images failed to load for various reasons, leaving blank spaces in our layout. After implementing error handling, the user experience was significantly improved.
Common Pitfalls and How to Overcome Them
Over the years, I’ve come across a few common pitfalls that developers often face. But fret not, there are ways to overcome them. Let’s go over a few of these:
Pitfall 1: Network Image not Displaying
One of the most common issues you might encounter is a network image not displaying. This often happens because network images require dimensions to be set explicitly. Otherwise, they won’t show up.
<Image source={{ uri: 'https://your-image-url.png' }} style={{ width: 100, height: 100 }} />
Always set a specific height and width for network images.
Pitfall 2: Large Images Causing the App to Slow Down
If you’re working with large images, you might notice that your app slows down. This happens because large images consume a lot of memory, which can lead to performance issues.
Consider resizing your images before displaying them. You could use a service like Imgix or Cloudinary to resize the images server-side, or use a library like react-native-image-resizer
for client-side resizing.
Pitfall 3: Images Take a Long Time to Load
Network latency can cause images to load slowly, especially if you’re fetching them from a server located far from the user.
Utilize caching and consider using a Content Delivery Network (CDN) to reduce latency. For more control over image caching and loading, you could use a library like react-native-fast-image
or expo-image
.
import FastImage from 'react-native-fast-image'
<FastImage
source={{ uri: 'https://your-image-url.png', priority: FastImage.priority.high }}
/>
Pitfall 4: App Crashes When Image Fails to Load
Without proper error handling, your app can crash or behave unexpectedly when an image fails to load.
Always implement error handling when working with network images. The onError
event handler of the Image
component allows you to gracefully handle such scenarios.
<Image
source={{uri: 'https://your-image-url.png'}}
onError={() => {
console.log('Failed to load the image');
}}
/>
Frequently Asked Questions
What types of images does React Native support?
React Native supports JPEG, PNG, BMP, GIF, and WebP formats for Android. For iOS, it supports JPEG, PNG, and GIF.
Why is my network image not showing up in React Native?
Network images require explicit height and width set. If you didn’t set a specific size, the image may not show up.
How can I improve image loading times in React Native?
You can improve image loading times by optimizing images, resizing them appropriately, and implementing caching. Consider using libraries like react-native-fast-image
for advanced control over caching and loading.
How do I handle errors when loading images in React Native?
React Native’s Image component has an onError
callback prop that can be used to handle image loading errors. You could use this to display an error message or a fallback image.
What is the difference between local and network images in React Native?
Local images are the ones that are included in your app’s bundle. They load quickly and their size is added to the overall size of your app. Network images are downloaded from a remote server. They don’t contribute to the size of your app, but they need to be downloaded, which might take some time and depend on the user’s network speed.
What is ‘caching’ and why is it important for images in React Native?
Caching means storing the image data locally after it’s loaded for the first time. This speeds up the loading times on subsequent renders, as the image doesn’t need to be fetched again from its source. This is especially beneficial for network images.
What are some common pitfalls when working with images in React Native?
Some common pitfalls include not setting a specific size for network images, not optimizing large images, not implementing caching, and not handling loading errors.
How does ‘resizeMode’ work in React Native?
The resizeMode
prop in React Native determines how the image should be resized when the frame doesn’t match the raw image dimensions. It accepts cover
, contain
, stretch
, repeat
, center
as values.
What are some good libraries for handling images in React Native?
Some popular libraries for handling images in React Native are react-native-fast-image
and expo-image
for advanced image loading and caching, and react-native-image-resizer
for resizing images.
How to align an image at the center in React Native?
You can align an image at the center in React Native using flexbox.
<View style={{ flex: 1, justifyContent: 'center', alignItems: 'center' }}>
<Image source={require('./image.png')} />
</View>
The Image will be centered horizontally and vertically in its parent View.
How to detect when an image has loaded in React Native?
You can use the onLoad
event, which is called when the image finishes loading. For example:
<Image source={{uri: 'https://your-image-url.png'}} onLoad={() => console.log('Image loaded')} />
How to show a Base64 image in React Native?
You can display a Base64 image by using the uri
property of the source prop. Here’s an example:
<Image source={{uri: 'data:image/png;base64,iVBORw0KGg....'}} />
Don’t forget to specify a height and a width, otherwise your image won’t be displayed!
How to make an image appear slowly in React Native?
You can use React Native’s Animation API or Reanimated to gradually change the opacity of an image, making it appear slowly. This animation is also called a “fade-in”.
import React, { useRef, useEffect } from 'react';
import { Animated, View, StyleSheet } from 'react-native';
const FadeInImage = () => {
const opacity = useRef(new Animated.Value(0)).current;
const onLoad = () => {
Animated.timing(opacity, {
toValue: 1,
duration: 2000,
useNativeDriver: true,
}).start();
};
return (
<View>
<Animated.Image
style={[styles.image, { opacity }]}
source={{ uri: 'https://your-image-url.jpg' }}
onLoad={onLoad}
/>
</View>
);
};
We define the onLoad
function that starts the fade-in animation, and pass it as a prop to the Image
component. When the image finishes loading, the onLoad
callback is triggered, starting the animation.
Check out the Animation API documentation for more information.
How to use SVG image in React Native?
React Native does not support SVG images out of the box. You need to use the SvgUri component from the library react-native-svg
. After installing the library, you can import SVG images and use them as React components.
<SvgUri source="https://your-image-url.svg" />
Resources and Further Reading
Here are some resources and further readings that can help you deepen your knowledge:
- Official React Native Documentation: A great place to start and always refer back to. You can learn more about the Image component and its props.
- React Native Fast Image Library: A great library for handling images, offering a rich feature set including caching, priority, and more. You can find the library here.
- React Native Image Resizer: This is a very useful library if you need to handle resizing on the client side. Check it out here.
- React Native School: A great platform with many useful articles and tutorials. Their guide on Handling Images in React Native is particularly insightful.
- React Native Community: The community is a great place to stay updated with the latest developments, ask questions, and interact with other React Native developers. You can join the React Native Community on GitHub.
- Egghead.io: This learning platform has many bite-sized videos on React Native, including image handling. You can check out their React Native courses here.
Wrapping Up
We’ve covered the essentials of displaying images in React Native, starting from the basics and diving deep into more advanced topics and common pitfalls. It’s essential to understand that images can significantly impact the performance and user experience of your application.
The exciting part about technology, and specifically React Native, is that it’s always evolving. As you continue to build more complex and diverse applications, I encourage you to keep exploring and learning. Stay updated with the latest developments in the community, experiment with new libraries and techniques, and most importantly, have fun coding!
Thank you for joining me on this journey to understand the essentials of displaying images in React Native. I hope you found this guide helpful, and I’m excited to see the amazing things you will build!
Don’t forget to share it with your friends and colleagues who are also learning React Native. Cheers!
Have a look at some of our other articles:
- How Long Does it Take to Learn React Native?
- The Beginner’s Guide to Styling in React Native
- React Native Essentials: The Ultimate Guide to Flexbox
- React Native Essentials: Getting started with React Navigation
- React Native Essentials: How to use Flatlist
- React Native Essentials: How to use TextInput
- 5 Common useEffect Mistakes Every Junior React Native Developer Should Avoid
- React Native Essentials: How to Handle Touch events with Pressable